In this article, we'll briefly go over how to setup OpenAI and integrate chat completions into your C# and .NET application.
Firstly, what are chat completions?
When interacting with ChatGPT or a similar LLM where you "chat" with it, you're using a type of chat completion system. A chat completion system allows the LLM to remember the context of your conversation with it so that it can respond accordingly. Chat completions use an API where for each chat response requested from the API, you must send the entire chat history with the request so the LLM can properly respond to it.
Click here to watch the full video on YouTube and subscribe!
All code samples are also available here on GitHub: https://github.com/AvironSoftware/dotnet-ai-examples/tree/main/OpenAITest
Setting up OpenAI to use their API
First, go to https://openai.com and create an account if you don't have one already.
Then, navigate to https:/ject name, then Manage Projects.

Click on Billing and ensure that you have credits available - if not, add some! :)
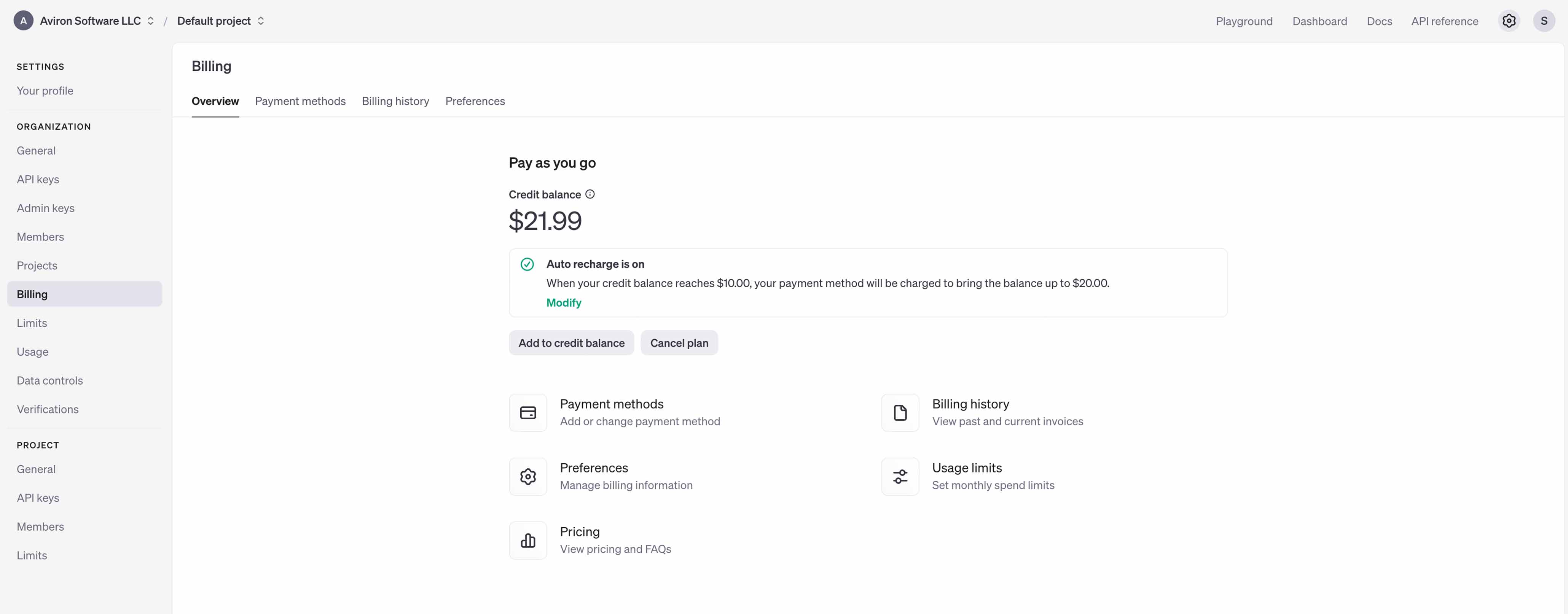
Click on API Keys and create a new API key for use with your API.
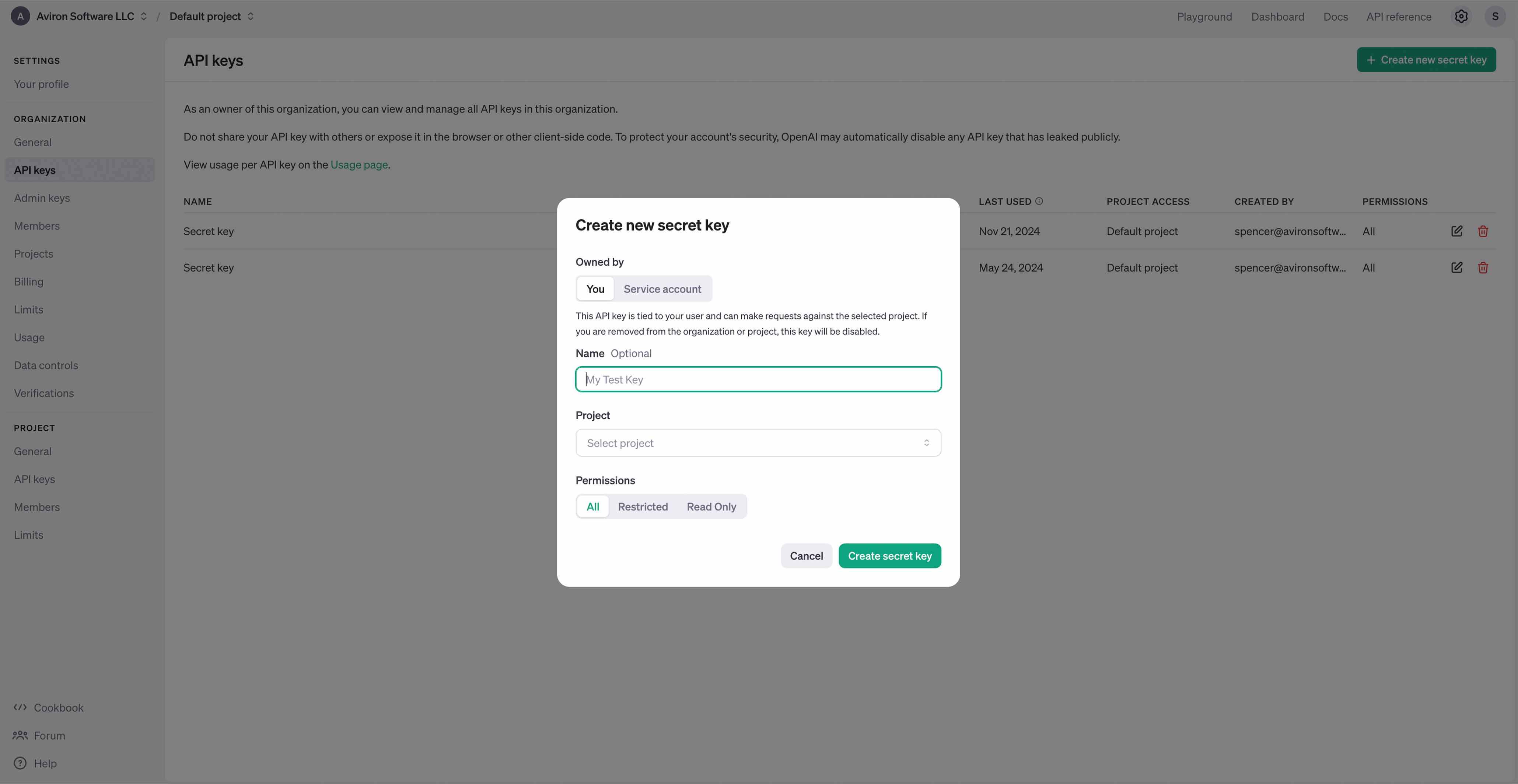
You're now ready to use the OpenAI API in your .NET app!
Connecting our C# app with OpenAI
Start with a fresh console app. You can create one using your IDE or the `dotnet` CLI:
dotnet new console
Firstly, install this NuGet package using your IDE or the dotnet CLI:
dotnet add package OpenAI
Import the proper classes like so:
using OpenAI.Chat;
Then you can declare the variables that will be used to authenticate you to the OpenAI API. You only need one in this case:
var openAIApiKey = "";
You can then declare your API clients like so (we're using gpt-4o in this example as our model):
var client = new ChatClient("gpt-4o", openAIApiKey);
Afterwards, you'll declare the container for all of your chat messages:
var messages = new List<ChatMessage>();
Finally, we'll create the loop that we'll use to interact with the OpenAI chat completions API.
We'll:
1. Read the line written by the user. (Exit the loop if the user writes "exit".)
2. Add it to the messages `List` declared above.
3. Send the entire message list to the API.
4. Read the response and print it to the console.
5. Add the messages to the messages list so it can be sent in subsequent requests.
while (true)
{
var line = Console.ReadLine();
if (line == "exit")
{
break;
}
messages.Add(new UserChatMessage(line));
var response = await client.CompleteChatAsync(messages);
var chatResponse = response.Value.Content.Last().Text;
Console.WriteLine(chatResponse);
messages.Add(new AssistantChatMessage(chatResponse));
}
Finally, run the solution, and if everything is working correctly, you should be able to chat with your API.
You can alter this example to do things like add a system message to change the behavior of the chat. For example, you can ask it to respond to all requests in a Southern accent!
var messages = new List<ChatMessage>
{
new SystemChatMessage("You have a Southern accent and are friendly!")
};
More Resources
Get The Code!
https://github.com/AvironSoftware/dotnet-ai-examples/tree/main/OpenAITest
OpenAI Pricing
https://openai.com/api/pricing/
Watch the Full Video on the Aviron Software YouTube Channel
Unlock the latest tech tips and insider insights on .NET, React, ASP.NET, React Native, Azure, and beyond with Aviron Software—your go-to source for mastering the tools shaping the future of development!
Looking to integrate AI & LLM features into your applications?
Aviron Software is the Official Software Consultancy founded by Spencer Schneidenbach. Aviron Software can align with your in-house team, or consultancy, to produce, test & publish custom software solutions.